mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
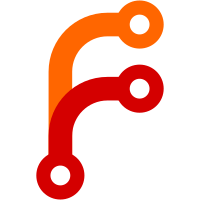
* Make sure reading the body of same JSON page request safe. It's not possible to read the body twice from fetch()'s response So, we've to clone the response before doing anything. * Fix tests.
63 lines
1.8 KiB
JavaScript
63 lines
1.8 KiB
JavaScript
/* global describe, it, expect */
|
|
import Router from '../../dist/lib/router/router'
|
|
|
|
describe('Router', () => {
|
|
const request = { clone: () => null }
|
|
describe('.prefetch()', () => {
|
|
it('should prefetch a given page', async () => {
|
|
const router = new Router('/', {})
|
|
const promise = Promise.resolve(request)
|
|
const route = 'routex'
|
|
router.doFetchRoute = (r) => {
|
|
expect(r).toBe(route)
|
|
return promise
|
|
}
|
|
await router.prefetch(route)
|
|
|
|
expect(router.fetchingRoutes[route]).toBe(promise)
|
|
})
|
|
|
|
it('should stop if it\'s prefetching already', async () => {
|
|
const router = new Router('/', {})
|
|
const route = 'routex'
|
|
router.fetchingRoutes[route] = Promise.resolve(request)
|
|
router.doFetchRoute = () => { throw new Error('Should not happen') }
|
|
await router.prefetch(route)
|
|
})
|
|
|
|
it('should only run two jobs at a time', async () => {
|
|
const router = new Router('/', {})
|
|
let count = 0
|
|
|
|
router.doFetchRoute = () => {
|
|
count++
|
|
return new Promise((resolve) => {})
|
|
}
|
|
|
|
router.prefetch('route1')
|
|
router.prefetch('route2')
|
|
router.prefetch('route3')
|
|
router.prefetch('route4')
|
|
|
|
await new Promise((resolve) => setTimeout(resolve, 50))
|
|
|
|
expect(count).toBe(2)
|
|
expect(Object.keys(router.fetchingRoutes)).toEqual(['route1', 'route2'])
|
|
})
|
|
|
|
it('should run all the jobs', async () => {
|
|
const router = new Router('/', {})
|
|
const routes = ['route1', 'route2', 'route3', 'route4']
|
|
|
|
router.doFetchRoute = () => Promise.resolve(request)
|
|
|
|
await router.prefetch(routes[0])
|
|
await router.prefetch(routes[1])
|
|
await router.prefetch(routes[2])
|
|
await router.prefetch(routes[3])
|
|
|
|
expect(Object.keys(router.fetchingRoutes)).toEqual(routes)
|
|
})
|
|
})
|
|
})
|