mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
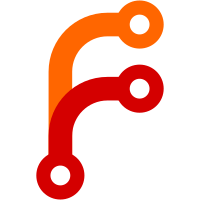
* Expose pages/_app.js * Add tests for _app and _document * Uncomment deprecation warnings * Add documentation for _app, improve documentation of _document * Update docs / test for _document * Add _document to client compiler in development * Add missing app.js to comment * Only warn once * Add url-deprecated error page * Combine tests * Yse same message for all methods of ‘props.url’ * Update docs around _app * Update documentation * Quotes * Update table of contents
90 lines
2.4 KiB
JavaScript
90 lines
2.4 KiB
JavaScript
import 'event-source-polyfill'
|
|
import webpackHotMiddlewareClient from 'webpack-hot-middleware/client?autoConnect=false&overlay=false&reload=true'
|
|
import Router from '../lib/router'
|
|
|
|
const {
|
|
__NEXT_DATA__: {
|
|
assetPrefix
|
|
}
|
|
} = window
|
|
|
|
export default () => {
|
|
webpackHotMiddlewareClient.setOptionsAndConnect({
|
|
path: `${assetPrefix}/_next/webpack-hmr`
|
|
})
|
|
|
|
const handlers = {
|
|
reload (route) {
|
|
if (route === '/_error') {
|
|
for (const r of Object.keys(Router.components)) {
|
|
const { err } = Router.components[r]
|
|
if (err) {
|
|
// reload all error routes
|
|
// which are expected to be errors of '/_error' routes
|
|
Router.reload(r)
|
|
}
|
|
}
|
|
return
|
|
}
|
|
|
|
// If the App component changes we have to reload the current route
|
|
if (route === '/_app') {
|
|
Router.reload(Router.route)
|
|
return
|
|
}
|
|
|
|
// Since _document is server only we need to reload the full page when it changes.
|
|
if (route === '/_document') {
|
|
window.location.reload()
|
|
return
|
|
}
|
|
|
|
Router.reload(route)
|
|
},
|
|
|
|
change (route) {
|
|
// If the App component changes we have to reload the current route
|
|
if (route === '/_app') {
|
|
Router.reload(Router.route)
|
|
return
|
|
}
|
|
|
|
// Since _document is server only we need to reload the full page when it changes.
|
|
if (route === '/_document') {
|
|
window.location.reload()
|
|
return
|
|
}
|
|
|
|
const { err, Component } = Router.components[route] || {}
|
|
|
|
if (err) {
|
|
// reload to recover from runtime errors
|
|
Router.reload(route)
|
|
}
|
|
|
|
if (Router.route !== route) {
|
|
// If this is a not a change for a currently viewing page.
|
|
// We don't need to worry about it.
|
|
return
|
|
}
|
|
|
|
if (!Component) {
|
|
// This only happens when we create a new page without a default export.
|
|
// If you removed a default export from a exising viewing page, this has no effect.
|
|
console.log(`Hard reloading due to no default component in page: ${route}`)
|
|
window.location.reload()
|
|
}
|
|
}
|
|
}
|
|
|
|
webpackHotMiddlewareClient.subscribe((obj) => {
|
|
const fn = handlers[obj.action]
|
|
if (fn) {
|
|
const data = obj.data || []
|
|
fn(...data)
|
|
} else {
|
|
throw new Error('Unexpected action ' + obj.action)
|
|
}
|
|
})
|
|
}
|