mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
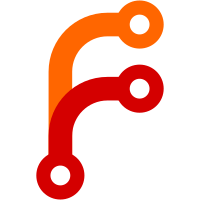
* Add with-context-api example * Change next dependency to canary and fix CounterProvider import
44 lines
842 B
JavaScript
44 lines
842 B
JavaScript
import React, { Component } from 'react'
|
|
|
|
/* First we will make a new context */
|
|
const CounterContext = React.createContext()
|
|
|
|
/* Then create a provider Component */
|
|
class CounterProvider extends Component {
|
|
state = {
|
|
count: 0
|
|
}
|
|
|
|
increase = () => {
|
|
this.setState({
|
|
count: this.state.count + 1
|
|
})
|
|
}
|
|
|
|
decrease = () => {
|
|
this.setState({
|
|
count: this.state.count - 1
|
|
})
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<CounterContext.Provider
|
|
value={{
|
|
count: this.state.count,
|
|
increase: this.increase,
|
|
decrease: this.decrease
|
|
}}
|
|
>
|
|
{this.props.children}
|
|
</CounterContext.Provider>
|
|
)
|
|
}
|
|
}
|
|
|
|
/* then make a consumer which will surface it */
|
|
const CounterConsumer = CounterContext.Consumer
|
|
|
|
export default CounterProvider
|
|
export { CounterConsumer }
|