mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
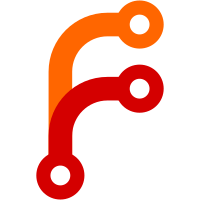
* Add .jsx extension * examples: add create-next-app (#3377) * examples: add create-next-app * fix with-typescript readme * Upgrading with-flow example to the latest flow-bin ver. 0.59.0 (#3337) For upgrading I used flow-upgrade module by https://yarnpkg.com/en/package/flow-upgrade * doc'd fs-routing option & added note on `passHref` (#3384) 2 changes: `passHref` - just added a cautionary note on the importance of `passHref`. We had a few days of no-href links on our site b/c we used a custom component instead of a raw `<a>` tag, and Google bot wasn't crawling our links (confirmed in Google cache). Hurt our SEO a bit, so I thought it was worth noting. `useFileSystemPublicRoutes` - this is mentioned in https://github.com/zeit/next.js/pull/914 , but it doesn't appear any doc was actually added. We use `next-routes`, and we were serving all the files in `/pages/` in addition to their route patterns (ie duplicate content), which can be a pain w/ SEO and duplicate content. * fix typo in readme.md (#3385) * Upgrade styled-jsx to v2.2.1 (#3358) * Pulled encoding to top of head (#3214) * Remove next.d.ts to use @types/next (#3297) * Add with-mobx-state-tree example (#3179) * Adapt with-mobx example for with-mobx-state-tree * Remove unnecessary lastUpdate parameter to show off snapshot * update readme * make other.js more closely mimic index.js * Upgrade styled-jsx to v2.2.1 Includes some bug fixes. * Fix linting * Make sure import that doesn’t end in .jsx works * Move tests * Show error when .js and .jsx both exist * Remove .jsx when importing from ‘path.jsx’ * Fixes * Get .jsx resolver back * Revert "Get .jsx resolver back" This reverts commit 6f76712caa400e6f41a6a32ff80189a95b194cce. * Revert "Revert "Get .jsx resolver back"" This reverts commit 69e592e86e53f28d0e1f78009196b76f2f831866. * Add remove .jsx to preset * Remove jsx resolver * Revert "Remove jsx resolver" This reverts commit 5e3ef1aca134de47657d91485809cd801e13329f. * Revert "Revert "Remove jsx resolver"" This reverts commit 8248e5066cff1c7e33dac2e5a88ffe6856e3fc4e. * Revert "Revert "Revert "Remove jsx resolver""" This reverts commit 2a6d418a227ea4e59874b0374628ef497e527c52. * Make 1 component not use .jsx
89 lines
2.4 KiB
JavaScript
89 lines
2.4 KiB
JavaScript
import { join, sep, parse } from 'path'
|
|
import fs from 'mz/fs'
|
|
import glob from 'glob-promise'
|
|
|
|
export default async function resolve (id) {
|
|
const paths = getPaths(id)
|
|
for (const p of paths) {
|
|
if (await isFile(p)) {
|
|
return p
|
|
}
|
|
}
|
|
|
|
const err = new Error(`Cannot find module ${id}`)
|
|
err.code = 'ENOENT'
|
|
throw err
|
|
}
|
|
|
|
export function resolveFromList (id, files) {
|
|
const paths = getPaths(id)
|
|
const set = new Set(files)
|
|
for (const p of paths) {
|
|
if (set.has(p)) return p
|
|
}
|
|
}
|
|
|
|
function getPaths (id) {
|
|
const i = sep === '/' ? id : id.replace(/\//g, sep)
|
|
|
|
if (i.slice(-3) === '.js') return [i]
|
|
if (i.slice(-4) === '.jsx') return [i]
|
|
if (i.slice(-5) === '.json') return [i]
|
|
|
|
if (i[i.length - 1] === sep) {
|
|
return [
|
|
i + 'index.js',
|
|
i + 'index.jsx',
|
|
i + 'index.json'
|
|
]
|
|
}
|
|
|
|
return [
|
|
i + '.js',
|
|
join(i, 'index.js'),
|
|
i + '.jsx',
|
|
join(i, 'index.jsx'),
|
|
i + '.json',
|
|
join(i, 'index.json')
|
|
]
|
|
}
|
|
|
|
async function isFile (p) {
|
|
let stat
|
|
try {
|
|
stat = await fs.stat(p)
|
|
} catch (err) {
|
|
if (err.code === 'ENOENT') return false
|
|
throw err
|
|
}
|
|
|
|
// We need the path to be case sensitive
|
|
const realpath = await getTrueFilePath(p)
|
|
if (p !== realpath) return false
|
|
|
|
return stat.isFile() || stat.isFIFO()
|
|
}
|
|
|
|
// This is based on the stackoverflow answer: http://stackoverflow.com/a/33139702/457224
|
|
// We assume we'll get properly normalized path names as p
|
|
async function getTrueFilePath (p) {
|
|
let fsPathNormalized = p
|
|
// OSX: HFS+ stores filenames in NFD (decomposed normal form) Unicode format,
|
|
// so we must ensure that the input path is in that format first.
|
|
if (process.platform === 'darwin') fsPathNormalized = fsPathNormalized.normalize('NFD')
|
|
|
|
// !! Windows: Curiously, the drive component mustn't be part of a glob,
|
|
// !! otherwise glob.sync() will invariably match nothing.
|
|
// !! Thus, we remove the drive component and instead pass it in as the 'cwd'
|
|
// !! (working dir.) property below.
|
|
var pathRoot = parse(fsPathNormalized).root
|
|
var noDrivePath = fsPathNormalized.slice(Math.max(pathRoot.length - 1, 0))
|
|
|
|
// Perform case-insensitive globbing (on Windows, relative to the drive /
|
|
// network share) and return the 1st match, if any.
|
|
// Fortunately, glob() with nocase case-corrects the input even if it is
|
|
// a *literal* path.
|
|
const result = await glob(noDrivePath, { nocase: true, cwd: pathRoot })
|
|
return result[0]
|
|
}
|