mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
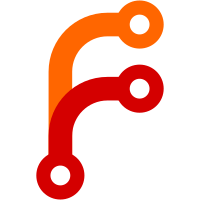
* With-Redux-example-update-request Hello Next.js, I’ve added an additional example to “With-Redux” and updated some of the original code to help illustrate to less inexperienced developers how to implement Redux with Next.js. The example is a simple counter to help reinforce how the client and server renderings work together. In addition I also updated some of the redux boilerplate code to help fully demonstrate how redux can be implemented when using is with Next.js Please contact me at spencer.bigum@gmail.com for further questions or anything else you might need. Thanks, Spencer * fixed listing issues: examples/with-redux * Updated code based on @impronunciable Feedback
36 lines
778 B
JavaScript
36 lines
778 B
JavaScript
import React, {Component} from 'react'
|
|
import { connect } from 'react-redux'
|
|
import { bindActionCreators } from 'redux'
|
|
import { addCount } from '../store'
|
|
|
|
class AddCount extends Component {
|
|
add = () => {
|
|
this.props.addCount()
|
|
}
|
|
|
|
render () {
|
|
const { count } = this.props
|
|
return (
|
|
<div>
|
|
<style jsx>{`
|
|
div {
|
|
padding: 0 0 20px 0;
|
|
}
|
|
`}</style>
|
|
<h1>AddCount: <span>{count}</span></h1>
|
|
<button onClick={this.add}>Add To Count</button>
|
|
</div>
|
|
)
|
|
}
|
|
}
|
|
|
|
const mapStateToProps = ({ count }) => ({ count })
|
|
|
|
const mapDispatchToProps = (dispatch) => {
|
|
return {
|
|
addCount: bindActionCreators(addCount, dispatch)
|
|
}
|
|
}
|
|
|
|
export default connect(mapStateToProps, mapDispatchToProps)(AddCount)
|