mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
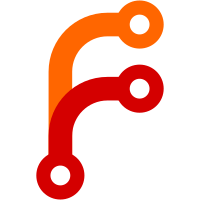
* add 'next' api * add render APIs * add 'as' prop to Link * check Accept header to serve json response * check if response was finished on getInitialProps call * move server/app to server/index * load webpack-hot-middleware-client by absolute path * server: options for testing * add tests * example: improve * server: make dir optional * fix client routing * add parameterized routing example * link: fix display url * Add custom-server-express example (#352) * Add custom-server-express example * Remove extraneous nexts in express routes defs * Update next config in server.js * Handle accept headers totally inside Next.js (#385) * Handle accept headers totally inside Next.js Now user doesn't need to handle it anymore. * Move json pages serving to /_next/pages base path. * Join paths correctly. * remove next/render
73 lines
1.7 KiB
JavaScript
73 lines
1.7 KiB
JavaScript
import React, { Component, PropTypes, Children } from 'react'
|
|
|
|
export default class Link extends Component {
|
|
static contextTypes = {
|
|
router: PropTypes.object
|
|
}
|
|
|
|
constructor (props) {
|
|
super(props)
|
|
this.linkClicked = this.linkClicked.bind(this)
|
|
}
|
|
|
|
linkClicked (e) {
|
|
if (e.target.nodeName === 'A' &&
|
|
(e.metaKey || e.ctrlKey || e.shiftKey || (e.nativeEvent && e.nativeEvent.which === 2))) {
|
|
// ignore click for new tab / new window behavior
|
|
return
|
|
}
|
|
|
|
const { href, scroll, as } = this.props
|
|
|
|
if (!isLocal(href)) {
|
|
// ignore click if it's outside our scope
|
|
return
|
|
}
|
|
|
|
e.preventDefault()
|
|
|
|
const route = as ? href : null
|
|
const url = as || href
|
|
|
|
// straight up redirect
|
|
this.context.router.push(route, url)
|
|
.then((success) => {
|
|
if (!success) return
|
|
if (scroll !== false) window.scrollTo(0, 0)
|
|
})
|
|
.catch((err) => {
|
|
if (this.props.onError) this.props.onError(err)
|
|
})
|
|
}
|
|
|
|
render () {
|
|
const children = Children.map(this.props.children, (child) => {
|
|
const props = {
|
|
onClick: this.linkClicked
|
|
}
|
|
|
|
const isAnchor = child && child.type === 'a'
|
|
|
|
// if child does not specify a href, specify it
|
|
// so that repetition is not needed by the user
|
|
if (!isAnchor || !('href' in child.props)) {
|
|
props.href = this.props.as || this.props.href
|
|
}
|
|
|
|
if (isAnchor) {
|
|
return React.cloneElement(child, props)
|
|
} else {
|
|
return <a {...props}>{child}</a>
|
|
}
|
|
})
|
|
|
|
return children[0]
|
|
}
|
|
}
|
|
|
|
export function isLocal (href) {
|
|
const origin = window.location.origin
|
|
return !/^https?:\/\//.test(href) ||
|
|
origin === href.substr(0, origin.length)
|
|
}
|