mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
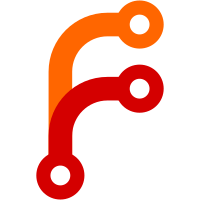
The mobx-state-tree examples (with and without typescript) pre-dated the next _app class and needed to be updated to use _app for persisting state across client-side navigation transitions. Also removed unneeded custom server class to better keep with the "show one feature per example" style of the next examples folder
46 lines
1.2 KiB
JavaScript
46 lines
1.2 KiB
JavaScript
import React from 'react'
|
|
import { Provider } from 'mobx-react'
|
|
import { getSnapshot } from 'mobx-state-tree'
|
|
import App, { Container } from 'next/app'
|
|
import { initializeStore } from '../stores/store'
|
|
|
|
export default class MyApp extends App {
|
|
static async getInitialProps ({ Component, router, ctx }) {
|
|
//
|
|
// Use getInitialProps as a step in the lifecycle when
|
|
// we can initialize our store
|
|
//
|
|
const isServer = (typeof window === 'undefined')
|
|
const store = initializeStore(isServer)
|
|
//
|
|
// Check whether the page being rendered by the App has a
|
|
// static getInitialProps method and if so call it
|
|
//
|
|
let pageProps = {}
|
|
if (Component.getInitialProps) {
|
|
pageProps = await Component.getInitialProps(ctx)
|
|
}
|
|
return {
|
|
initialState: getSnapshot(store),
|
|
isServer,
|
|
pageProps
|
|
}
|
|
}
|
|
|
|
constructor (props) {
|
|
super(props)
|
|
this.store = initializeStore(props.isServer, props.initialState)
|
|
}
|
|
|
|
render () {
|
|
const { Component, pageProps } = this.props
|
|
return (
|
|
<Container>
|
|
<Provider store={this.store}>
|
|
<Component {...pageProps} />
|
|
</Provider>
|
|
</Container>
|
|
)
|
|
}
|
|
}
|