mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
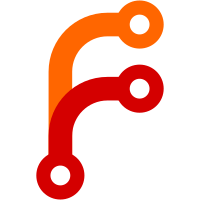
Change file name format on folder lib like /examples/with-apollo and /examples/with-apollo-and-redux
50 lines
1.3 KiB
JavaScript
50 lines
1.3 KiB
JavaScript
import React from 'react'
|
|
import cookie from 'cookie'
|
|
import { withApollo, compose } from 'react-apollo'
|
|
|
|
import withData from '../lib/withData'
|
|
import redirect from '../lib/redirect'
|
|
import checkLoggedIn from '../lib/checkLoggedIn'
|
|
|
|
class Index extends React.Component {
|
|
static async getInitialProps (context, apolloClient) {
|
|
const { loggedInUser } = await checkLoggedIn(context, apolloClient)
|
|
|
|
if (!loggedInUser.user) {
|
|
// If not signed in, send them somewhere more useful
|
|
redirect(context, '/signin')
|
|
}
|
|
|
|
return { loggedInUser }
|
|
}
|
|
|
|
signout = () => {
|
|
document.cookie = cookie.serialize('token', '', {
|
|
maxAge: -1 // Expire the cookie immediately
|
|
})
|
|
|
|
// Force a reload of all the current queries now that the user is
|
|
// logged in, so we don't accidentally leave any state around.
|
|
this.props.client.resetStore().then(() => {
|
|
// Redirect to a more useful page when signed out
|
|
redirect({}, '/signin')
|
|
})
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<div>
|
|
Hello {this.props.loggedInUser.user.name}!<br />
|
|
<button onClick={this.signout}>Sign out</button>
|
|
</div>
|
|
)
|
|
}
|
|
};
|
|
|
|
export default compose(
|
|
// withData gives us server-side graphql queries before rendering
|
|
withData,
|
|
// withApollo exposes `this.props.client` used when logging out
|
|
withApollo
|
|
)(Index)
|