mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
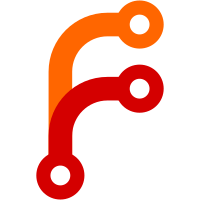
**Changes:** - Fixed "was character fetched on server" message by properly passing `isServer`. - Stop fetching if there was an error (currently it keeps sending requests to the same endpoint every 3 sec) **Related:** - https://github.com/zeit/next.js/pull/4818 - https://github.com/zeit/next.js/issues/4724
44 lines
1.1 KiB
JavaScript
44 lines
1.1 KiB
JavaScript
import React from 'react'
|
|
import { connect } from 'react-redux'
|
|
|
|
const CharacterInfo = ({ character, error, fetchCharacter, isFetchedOnServer = false }) => (
|
|
<div className='CharacterInfo'>
|
|
{
|
|
error ? <p>We encountered and error.</p>
|
|
: <article>
|
|
<h3>Character: {character.name}</h3>
|
|
<p>birth year: {character.birth_year}</p>
|
|
<p>gender: {character.gender}</p>
|
|
<p>skin color: {character.skin_color}</p>
|
|
<p>eye color: {character.eye_color}</p>
|
|
</article>
|
|
|
|
}
|
|
<p>
|
|
(was character fetched on server? -{' '}
|
|
<b>{isFetchedOnServer.toString()})</b>
|
|
</p>
|
|
<style jsx>{`
|
|
article {
|
|
background-color: #528CE0;
|
|
border-radius: 15px;
|
|
padding: 15px;
|
|
width: 250px;
|
|
margin: 15px 0;
|
|
color: white;
|
|
}
|
|
button {
|
|
margin-right: 10px;
|
|
}
|
|
`}</style>
|
|
</div>
|
|
)
|
|
|
|
export default connect(
|
|
state => ({
|
|
character: state.character,
|
|
error: state.error,
|
|
isFetchedOnServer: state.isFetchedOnServer
|
|
})
|
|
)(CharacterInfo)
|