mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
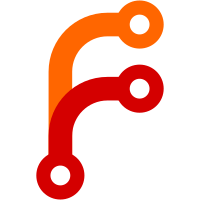
* With-Redux-example-update-request Hello Next.js, I’ve added an additional example to “With-Redux” and updated some of the original code to help illustrate to less inexperienced developers how to implement Redux with Next.js. The example is a simple counter to help reinforce how the client and server renderings work together. In addition I also updated some of the redux boilerplate code to help fully demonstrate how redux can be implemented when using is with Next.js Please contact me at spencer.bigum@gmail.com for further questions or anything else you might need. Thanks, Spencer * fixed listing issues: examples/with-redux * Updated code based on @impronunciable Feedback
38 lines
894 B
JavaScript
38 lines
894 B
JavaScript
import React from 'react'
|
|
import { bindActionCreators } from 'redux'
|
|
import { initStore, startClock, addCount, serverRenderClock } from '../store'
|
|
import withRedux from 'next-redux-wrapper'
|
|
import Page from '../components/Page'
|
|
|
|
class Counter extends React.Component {
|
|
static getInitialProps ({ store, isServer }) {
|
|
store.dispatch(serverRenderClock(isServer))
|
|
store.dispatch(addCount())
|
|
|
|
return { isServer }
|
|
}
|
|
|
|
componentDidMount () {
|
|
this.timer = this.props.startClock()
|
|
}
|
|
|
|
componentWillUnmount () {
|
|
clearInterval(this.timer)
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<Page title='Index Page' linkTo='/other' />
|
|
)
|
|
}
|
|
}
|
|
|
|
const mapDispatchToProps = (dispatch) => {
|
|
return {
|
|
addCount: bindActionCreators(addCount, dispatch),
|
|
startClock: bindActionCreators(startClock, dispatch)
|
|
}
|
|
}
|
|
|
|
export default withRedux(initStore, null, mapDispatchToProps)(Counter)
|