mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
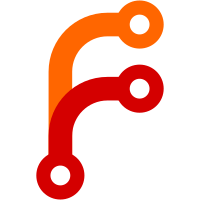
* Added next bootstrap command for starting a new project * renamed bootstrap to init and check we are not in a dir called pages * Removed extra empty line
57 lines
1.2 KiB
JavaScript
Executable file
57 lines
1.2 KiB
JavaScript
Executable file
#!/usr/bin/env node
|
|
import { resolve, join, basename } from 'path'
|
|
import parseArgs from 'minimist'
|
|
import { exists, writeFile, mkdir } from 'mz/fs'
|
|
|
|
const argv = parseArgs(process.argv.slice(2), {
|
|
alias: {
|
|
h: 'help'
|
|
},
|
|
boolean: ['h']
|
|
})
|
|
|
|
const dir = resolve(argv._[0] || '.')
|
|
|
|
exists(join(dir, 'package.json'))
|
|
.then(async present => {
|
|
if (basename(dir) === 'pages') {
|
|
console.warn('Your root directory is named "pages". This looks suspicious. You probably want to go one directory up.')
|
|
return
|
|
}
|
|
|
|
if (!present) {
|
|
await writeFile(join(dir, 'package.json'), basePackage)
|
|
}
|
|
|
|
if (!await exists(join(dir, 'static'))) {
|
|
await mkdir(join(dir, 'static'))
|
|
}
|
|
|
|
if (!await exists(join(dir, 'pages'))) {
|
|
await mkdir(join(dir, 'pages'))
|
|
await writeFile(join(dir, 'pages', 'index.js'), basePage)
|
|
}
|
|
})
|
|
.catch((err) => {
|
|
console.error(err)
|
|
exit(1)
|
|
})
|
|
|
|
const basePackage = `{
|
|
"name": "my-app",
|
|
"description": "my app",
|
|
"dependencies": {
|
|
"next": "latest"
|
|
},
|
|
"scripts": {
|
|
"dev": "next",
|
|
"build": "next build",
|
|
"start": "next start"
|
|
}
|
|
}`
|
|
|
|
const basePage =`
|
|
import React from 'react'
|
|
export default () => <p>Hello, world</p>
|
|
`
|