mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
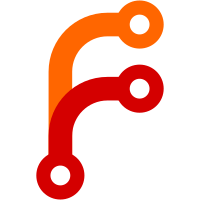
I had some trouble to get server side rendering with the AWSAppSyncClient working. I finally found a solution in https://github.com/awslabs/aws-mobile-appsync-sdk-js/issues/82 but it might be worth to share it here as well. Instead of adding a big code block to each file I'll just refer to this Pull Request. ______ In case you want to use the `AWSAppSyncClient` you just need to replace the `create()` function with this function: ```jsx import AWSAppSyncClient from 'aws-appsync'; import { AUTH_TYPE } from 'aws-appsync/lib/link/auth-link'; function create(initialState) { const client = new AWSAppSyncClient({ url: AWS_AppSync.graphqlEndpoint, region: AWS_AppSync.region, auth: { type: AUTH_TYPE.API_KEY, apiKey: AWS_AppSync.apiKey, // Amazon Cognito Federated Identities using AWS Amplify //credentials: () => Auth.currentCredentials(), // Amazon Cognito user pools using AWS Amplify // type: AUTH_TYPE.AMAZON_COGNITO_USER_POOLS, // jwtToken: async () => (await Auth.currentSession()).getIdToken().getJwtToken(), }, disableOffline: true, }, { cache: new InMemoryCache().restore(initialState || {}), ssrMode: true }); return client; } ```
52 lines
1.5 KiB
JavaScript
52 lines
1.5 KiB
JavaScript
import { ApolloClient, InMemoryCache } from 'apollo-boost'
|
|
import { createHttpLink } from 'apollo-link-http'
|
|
import { setContext } from 'apollo-link-context'
|
|
import fetch from 'isomorphic-unfetch'
|
|
|
|
let apolloClient = null
|
|
|
|
// Polyfill fetch() on the server (used by apollo-client)
|
|
if (!process.browser) {
|
|
global.fetch = fetch
|
|
}
|
|
|
|
function create (initialState, { getToken }) {
|
|
const httpLink = createHttpLink({
|
|
uri: 'https://api.graph.cool/simple/v1/cj5geu3slxl7t0127y8sity9r',
|
|
credentials: 'same-origin'
|
|
})
|
|
|
|
const authLink = setContext((_, { headers }) => {
|
|
const token = getToken()
|
|
return {
|
|
headers: {
|
|
...headers,
|
|
authorization: token ? `Bearer ${token}` : ''
|
|
}
|
|
}
|
|
})
|
|
|
|
// Check out https://github.com/zeit/next.js/pull/4611 if you want to use the AWSAppSyncClient
|
|
return new ApolloClient({
|
|
connectToDevTools: process.browser,
|
|
ssrMode: !process.browser, // Disables forceFetch on the server (so queries are only run once)
|
|
link: authLink.concat(httpLink),
|
|
cache: new InMemoryCache().restore(initialState || {})
|
|
})
|
|
}
|
|
|
|
export default function initApollo (initialState, options) {
|
|
// Make sure to create a new client for every server-side request so that data
|
|
// isn't shared between connections (which would be bad)
|
|
if (!process.browser) {
|
|
return create(initialState, options)
|
|
}
|
|
|
|
// Reuse client on the client-side
|
|
if (!apolloClient) {
|
|
apolloClient = create(initialState, options)
|
|
}
|
|
|
|
return apolloClient
|
|
}
|