mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
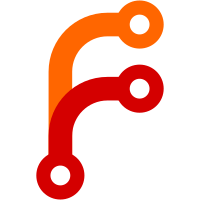
* Add minimal apollo example * Update apollo example README * Update apollo example demo link in README * Fix button styles * Fix show more button * Alias demo url * Include the data field on the Apollo store when hydrating * Revert * Include the data field on the Apollo store when hydrating per tpreusse's suggestion. * Add example to faq section in README * Sort by newest; Add active state to buttons * Make optimization suggestions * Use process.browser; inline props * Pass wrapped component's initial props into component heirarchy if they exist * Remove unnecessary sorting of array * Update Apollo example * Remove trailing comma * Update reduxRootKey * Remove unnecessary babelrc * Update with-apollo example - Remove use of deprecated 'reduxRootKey' option - Add loading indicator inside pagination button * Fix with-apollo example pagination; Pass initialState to ApolloClient * Split apollo example into two (one with and without Redux integration) * Rename createClient private function to _initClient * Set initialState default parameter inside initClient function * Remove redux dep from with-apollo example
51 lines
1.2 KiB
JavaScript
51 lines
1.2 KiB
JavaScript
import 'isomorphic-fetch'
|
|
import React from 'react'
|
|
import { ApolloProvider, getDataFromTree } from 'react-apollo'
|
|
import { initClient } from './initClient'
|
|
|
|
export default (Component) => (
|
|
class extends React.Component {
|
|
static async getInitialProps (ctx) {
|
|
const headers = ctx.req ? ctx.req.headers : {}
|
|
const client = initClient(headers)
|
|
|
|
const props = {
|
|
url: { query: ctx.query, pathname: ctx.pathname },
|
|
...await (Component.getInitialProps ? Component.getInitialProps(ctx) : {})
|
|
}
|
|
|
|
if (!process.browser) {
|
|
const app = (
|
|
<ApolloProvider client={client}>
|
|
<Component {...props} />
|
|
</ApolloProvider>
|
|
)
|
|
await getDataFromTree(app)
|
|
}
|
|
|
|
return {
|
|
initialState: {
|
|
apollo: {
|
|
data: client.getInitialState().data
|
|
}
|
|
},
|
|
headers,
|
|
...props
|
|
}
|
|
}
|
|
|
|
constructor (props) {
|
|
super(props)
|
|
this.client = initClient(this.props.headers, this.props.initialState)
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<ApolloProvider client={this.client}>
|
|
<Component {...this.props} />
|
|
</ApolloProvider>
|
|
)
|
|
}
|
|
}
|
|
)
|