mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
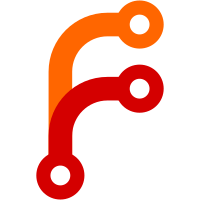
* Fix apollo warning and error when upvoting post * Fix apollo warning and error when upvoting post in apollo-redux example as well
57 lines
1.2 KiB
JavaScript
57 lines
1.2 KiB
JavaScript
import React from 'react'
|
|
import { gql, graphql } from 'react-apollo'
|
|
|
|
function PostUpvoter ({ upvote, votes, id }) {
|
|
return (
|
|
<button onClick={() => upvote(id, votes + 1)}>
|
|
{votes}
|
|
<style jsx>{`
|
|
button {
|
|
background-color: transparent;
|
|
border: 1px solid #e4e4e4;
|
|
color: #000;
|
|
}
|
|
button:active {
|
|
background-color: transparent;
|
|
}
|
|
button:before {
|
|
align-self: center;
|
|
border-color: transparent transparent #000000 transparent;
|
|
border-style: solid;
|
|
border-width: 0 4px 6px 4px;
|
|
content: "";
|
|
height: 0;
|
|
margin-right: 5px;
|
|
width: 0;
|
|
}
|
|
`}</style>
|
|
</button>
|
|
)
|
|
}
|
|
|
|
const upvotePost = gql`
|
|
mutation updatePost($id: ID!, $votes: Int) {
|
|
updatePost(id: $id, votes: $votes) {
|
|
id
|
|
__typename
|
|
votes
|
|
}
|
|
}
|
|
`
|
|
|
|
export default graphql(upvotePost, {
|
|
props: ({ ownProps, mutate }) => ({
|
|
upvote: (id, votes) => mutate({
|
|
variables: { id, votes },
|
|
optimisticResponse: {
|
|
__typename: 'Mutation',
|
|
updatePost: {
|
|
__typename: 'Post',
|
|
id: ownProps.id,
|
|
votes: ownProps.votes + 1
|
|
}
|
|
}
|
|
})
|
|
})
|
|
})(PostUpvoter)
|