mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
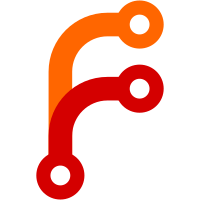
* display runtime errors by error-debug * server: fix status * render Error component on client error * server: render runtime errors of error template * server: handle errors of error template on render404 * server: add a comment * server: refactor renderJSON * recover from runtime errors * _error: check if xhr exists * _error: improve client error * _error: improve error message
87 lines
2.2 KiB
JavaScript
87 lines
2.2 KiB
JavaScript
import { join } from 'path'
|
|
import { parse } from 'url'
|
|
import { createElement } from 'react'
|
|
import { renderToString, renderToStaticMarkup } from 'react-dom/server'
|
|
import { renderStatic } from 'glamor/server'
|
|
import requireModule from './require'
|
|
import read from './read'
|
|
import getConfig from './config'
|
|
import Router from '../lib/router'
|
|
import Document from '../lib/document'
|
|
import Head, {defaultHead} from '../lib/head'
|
|
import App from '../lib/app'
|
|
|
|
export async function render (url, ctx = {}, {
|
|
dir = process.cwd(),
|
|
dev = false,
|
|
staticMarkup = false
|
|
} = {}) {
|
|
const path = getPath(url)
|
|
const mod = await requireModule(join(dir, '.next', 'dist', 'pages', path))
|
|
const Component = mod.default || mod
|
|
|
|
const [
|
|
props,
|
|
component,
|
|
errorComponent
|
|
] = await Promise.all([
|
|
Component.getInitialProps ? Component.getInitialProps(ctx) : {},
|
|
read(join(dir, '.next', 'bundles', 'pages', path)),
|
|
read(join(dir, '.next', 'bundles', 'pages', dev ? '_error-debug' : '_error'))
|
|
])
|
|
|
|
const { html, css, ids } = renderStatic(() => {
|
|
const app = createElement(App, {
|
|
Component,
|
|
props,
|
|
router: new Router(ctx.req ? ctx.req.url : url)
|
|
})
|
|
|
|
return (staticMarkup ? renderToStaticMarkup : renderToString)(app)
|
|
})
|
|
|
|
const head = Head.rewind() || defaultHead()
|
|
const config = await getConfig(dir)
|
|
|
|
const doc = createElement(Document, {
|
|
html,
|
|
head,
|
|
css,
|
|
data: {
|
|
component,
|
|
errorComponent,
|
|
props,
|
|
ids: ids,
|
|
err: (ctx.err && dev) ? errorToJSON(ctx.err) : null
|
|
},
|
|
dev,
|
|
staticMarkup,
|
|
cdn: config.cdn
|
|
})
|
|
|
|
return '<!DOCTYPE html>' + renderToStaticMarkup(doc)
|
|
}
|
|
|
|
export async function renderJSON (url, { dir = process.cwd() } = {}) {
|
|
const path = getPath(url)
|
|
const component = await read(join(dir, '.next', 'bundles', 'pages', path))
|
|
return { component }
|
|
}
|
|
|
|
export function errorToJSON (err) {
|
|
const { name, message, stack } = err
|
|
const json = { name, message, stack }
|
|
|
|
if (name === 'ModuleBuildError') {
|
|
// webpack compilation error
|
|
const { module: { rawRequest } } = err
|
|
json.module = { rawRequest }
|
|
}
|
|
|
|
return json
|
|
}
|
|
|
|
function getPath (url) {
|
|
return parse(url || '/').pathname.replace(/\.json$/, '')
|
|
}
|