mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
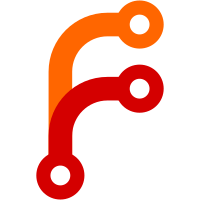
The `initialNow` prop is used to avoid content mismatches when Universal/SSR apps render date values using components like `<FormattedRelative>`. If this value is created in `render()`, then the server will generate it and then the client will also generate it during hydration / initial render, resulting in two different values and content mismatches like: > Warning: Text content did not match. Server: "in 1,741,545 seconds" Client: "in 1,741,543 seconds" If the value is instead generated in `getInitialProps`, then the client's initial rendering will match because it will use the same value sent down by the server.
43 lines
1.4 KiB
JavaScript
43 lines
1.4 KiB
JavaScript
import App, { Container } from 'next/app'
|
|
import React from 'react'
|
|
import { IntlProvider, addLocaleData } from 'react-intl'
|
|
|
|
// Register React Intl's locale data for the user's locale in the browser. This
|
|
// locale data was added to the page by `pages/_document.js`. This only happens
|
|
// once, on initial page load in the browser.
|
|
if (typeof window !== 'undefined' && window.ReactIntlLocaleData) {
|
|
Object.keys(window.ReactIntlLocaleData).forEach((lang) => {
|
|
addLocaleData(window.ReactIntlLocaleData[lang])
|
|
})
|
|
}
|
|
|
|
export default class MyApp extends App {
|
|
static async getInitialProps ({ Component, router, ctx }) {
|
|
let pageProps = {}
|
|
|
|
if (Component.getInitialProps) {
|
|
pageProps = await Component.getInitialProps(ctx)
|
|
}
|
|
|
|
// Get the `locale` and `messages` from the request object on the server.
|
|
// In the browser, use the same values that the server serialized.
|
|
const { req } = ctx
|
|
const { locale, messages } = req || window.__NEXT_DATA__.props
|
|
const initialNow = Date.now()
|
|
|
|
return { pageProps, locale, messages, initialNow }
|
|
}
|
|
|
|
render () {
|
|
const { Component, pageProps, locale, messages, initialNow } = this.props
|
|
|
|
return (
|
|
<Container>
|
|
<IntlProvider locale={locale} messages={messages} initialNow={initialNow}>
|
|
<Component {...pageProps} />
|
|
</IntlProvider>
|
|
</Container>
|
|
)
|
|
}
|
|
}
|