mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
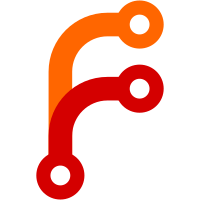
* Move route loading logic to a common place. * Add router events. * Add EventEmitter's core API methods. * Add example app for loading events and docs. * Fix some typos. * Get rid of Router.ready() * Remove events package. It's already shipping with webpack. * Handling aborting properly. * Expose simple attribute based events listener API. Removed the proposed event listener API from the public API. * Remove error logged when there's an abort error. There are many other ways to capture this error. So, it doesn't look nice to print this always. * Change router events to pass only the current URL as arguments. * Add a section about Cancelled Routes to README.
31 lines
874 B
JavaScript
31 lines
874 B
JavaScript
import React from 'react'
|
|
import Head from 'next/head'
|
|
import Link from 'next/link'
|
|
import NProgress from 'nprogress'
|
|
import Router from 'next/router'
|
|
|
|
Router.onRouteChangeStart = (url) => {
|
|
console.log(`Loading: ${url}`)
|
|
NProgress.start()
|
|
}
|
|
Router.onRouteChangeComplete = () => NProgress.done()
|
|
Router.onRouteChangeError = () => NProgress.done()
|
|
|
|
const linkStyle = {
|
|
margin: '0 10px 0 0'
|
|
}
|
|
|
|
export default () => (
|
|
<div style={{ marginBottom: 20 }}>
|
|
<Head>
|
|
{/* Import CSS for nprogress */}
|
|
<link rel='stylesheet' type='text/css' href='/static/nprogress.css' />
|
|
</Head>
|
|
|
|
<Link href='/'><a style={linkStyle}>Home</a></Link>
|
|
<Link href='/about'><a style={linkStyle}>About</a></Link>
|
|
<Link href='/forever'><a style={linkStyle}>Forever</a></Link>
|
|
<Link href='/non-existing'><a style={linkStyle}>Non Existing Page</a></Link>
|
|
</div>
|
|
)
|