mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
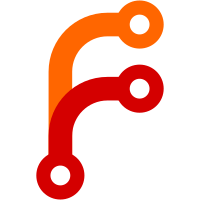
This patch adds an example of a Next.js app using analytics. A custom document injects the [Segment](https://segment.com) bootstrapping snippet into the `<head>`, allowing "page" and "track" calls to be made. An issue came up in CNA asking how we handle this in our apps (see https://github.com/segmentio/create-next-app/issues/24), so I figure an "official example" could help. NOTE: I am affiliated with Segment.
45 lines
1.1 KiB
JavaScript
45 lines
1.1 KiB
JavaScript
import React from 'react'
|
|
import Document, { Head, Main, NextScript } from 'next/document'
|
|
import * as snippet from '@segment/snippet'
|
|
|
|
const {
|
|
// This write key is associated with https://segment.com/nextjs-example/sources/nextjs.
|
|
ANALYTICS_WRITE_KEY = 'NPsk1GimHq09s7egCUlv7D0tqtUAU5wa',
|
|
NODE_ENV = 'development'
|
|
} = process.env
|
|
|
|
export default class extends Document {
|
|
static getInitialProps ({ renderPage }) {
|
|
const { html, head, errorHtml, chunks } = renderPage()
|
|
return { html, head, errorHtml, chunks }
|
|
}
|
|
|
|
renderSnippet () {
|
|
const opts = {
|
|
apiKey: ANALYTICS_WRITE_KEY,
|
|
page: true // Set this to `false` if you want to manually fire `analytics.page()` from within your pages.
|
|
}
|
|
|
|
if (NODE_ENV === 'development') {
|
|
return snippet.max(opts)
|
|
}
|
|
|
|
return snippet.min(opts)
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<html>
|
|
<Head>
|
|
{/* Inject the Segment snippet into the <head> of the document */}
|
|
<script dangerouslySetInnerHTML={{ __html: this.renderSnippet() }} />
|
|
</Head>
|
|
<body>
|
|
<Main />
|
|
<NextScript />
|
|
</body>
|
|
</html>
|
|
)
|
|
}
|
|
}
|