mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
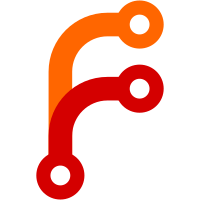
* Use file hashes instead of BUILD_ID. Now JSON pages also not prefixed with a hash and doesn't support immutable caching. Instead it supports Etag bases caching. * Remove appUpdated Router Events hook. Becuase now we don't need it because there's no buildId validation. * Remove buildId generation. * Turn off hash checks in the dev mode. * Update tests. * Revert "Remove buildId generation." This reverts commit fdd36a5a0a307becdbd1d85ae3881b3a15b03d26. * Bring back the buildId validation. * Handle buildId validation only in production. * Add BUILD_ID to path again. * Remove duplicate immutable header. * Fix tests.
65 lines
1.7 KiB
JavaScript
65 lines
1.7 KiB
JavaScript
import { tmpdir } from 'os'
|
|
import { join } from 'path'
|
|
import fs from 'mz/fs'
|
|
import uuid from 'uuid'
|
|
import del from 'del'
|
|
import webpack from './webpack'
|
|
import replaceCurrentBuild from './replace'
|
|
|
|
export default async function build (dir) {
|
|
const buildDir = join(tmpdir(), uuid.v4())
|
|
const compiler = await webpack(dir, { buildDir })
|
|
|
|
try {
|
|
const webpackStats = await runCompiler(compiler)
|
|
await writeBuildStats(buildDir, webpackStats)
|
|
await writeBuildId(buildDir)
|
|
} catch (err) {
|
|
console.error(`> Failed to build on ${buildDir}`)
|
|
throw err
|
|
}
|
|
|
|
await replaceCurrentBuild(dir, buildDir)
|
|
|
|
// no need to wait
|
|
del(buildDir, { force: true })
|
|
}
|
|
|
|
function runCompiler (compiler) {
|
|
return new Promise((resolve, reject) => {
|
|
compiler.run((err, stats) => {
|
|
if (err) return reject(err)
|
|
|
|
const jsonStats = stats.toJson()
|
|
|
|
if (jsonStats.errors.length > 0) {
|
|
const error = new Error(jsonStats.errors[0])
|
|
error.errors = jsonStats.errors
|
|
error.warnings = jsonStats.warnings
|
|
return reject(error)
|
|
}
|
|
|
|
resolve(jsonStats)
|
|
})
|
|
})
|
|
}
|
|
|
|
async function writeBuildStats (dir, webpackStats) {
|
|
const chunkHashMap = {}
|
|
webpackStats.chunks
|
|
// We are not interested about pages
|
|
.filter(({ files }) => !/^bundles/.test(files[0]))
|
|
.forEach(({ hash, files }) => {
|
|
chunkHashMap[files[0]] = { hash }
|
|
})
|
|
|
|
const buildStatsPath = join(dir, '.next', 'build-stats.json')
|
|
await fs.writeFile(buildStatsPath, JSON.stringify(chunkHashMap), 'utf8')
|
|
}
|
|
|
|
async function writeBuildId (dir) {
|
|
const buildIdPath = join(dir, '.next', 'BUILD_ID')
|
|
const buildId = uuid.v4()
|
|
await fs.writeFile(buildIdPath, buildId, 'utf8')
|
|
}
|