mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
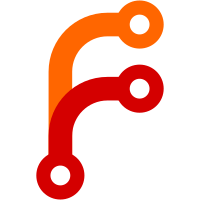
* [example] with-apollo-and-redux-saga - Using Apollo to get GraphQL Data? Dope. - Using Redux Saga to do other stuff outside of that? Cool. - Nary the two shall meet? Most likely. 😀️ This is a breakout of #3463 where we were combining Apollo and Redux. This may not be an example that gets a PR. Why? Well, the examples are meant to pick and choose and combine yourself. At least I believe, and this is basically a combination of two examples (`with-apollo` and `with-redux-saga`) with some reworking. **pages/**: `index`: withReduxSaga() `about`: () `blog/index`: withReduxSaga(withApollo()) `blog/entry`: withApollo() * [refactor] fix lint (again), remove superfluous calls * [fix] package.json: with-apollo-and-redux-saga Updated the `name` and made sure `es6-promise` was in dependencies * [refactor] remove semi-colons in clock/sagas * [refactor] remove old migration code
72 lines
1.6 KiB
JavaScript
72 lines
1.6 KiB
JavaScript
import { graphql } from 'react-apollo'
|
|
import gql from 'graphql-tag'
|
|
import { allPosts, allPostsQueryVars } from './PostList'
|
|
|
|
function Submit ({ createPost }) {
|
|
function handleSubmit (event) {
|
|
event.preventDefault()
|
|
|
|
const form = event.target
|
|
|
|
const formData = new window.FormData(form)
|
|
createPost(formData.get('title'), formData.get('url'))
|
|
|
|
form.reset()
|
|
}
|
|
|
|
return (
|
|
<form onSubmit={handleSubmit}>
|
|
<h1>Submit</h1>
|
|
<input placeholder='title' name='title' type='text' required />
|
|
<input placeholder='url' name='url' type='url' required />
|
|
<button type='submit'>Submit</button>
|
|
<style jsx>{`
|
|
form {
|
|
border-bottom: 1px solid #ececec;
|
|
padding-bottom: 20px;
|
|
margin-bottom: 20px;
|
|
}
|
|
input {
|
|
display: block;
|
|
margin-bottom: 10px;
|
|
}
|
|
`}</style>
|
|
</form>
|
|
)
|
|
}
|
|
|
|
const createPost = gql`
|
|
mutation createPost($title: String!, $url: String!) {
|
|
createPost(title: $title, url: $url) {
|
|
id
|
|
title
|
|
votes
|
|
url
|
|
createdAt
|
|
}
|
|
}
|
|
`
|
|
|
|
export default graphql(createPost, {
|
|
props: ({ mutate }) => ({
|
|
createPost: (title, url) =>
|
|
mutate({
|
|
variables: { title, url },
|
|
update: (proxy, { data: { createPost } }) => {
|
|
const data = proxy.readQuery({
|
|
query: allPosts,
|
|
variables: allPostsQueryVars
|
|
})
|
|
proxy.writeQuery({
|
|
query: allPosts,
|
|
data: {
|
|
...data,
|
|
allPosts: [createPost, ...data.allPosts]
|
|
},
|
|
variables: allPostsQueryVars
|
|
})
|
|
}
|
|
})
|
|
})
|
|
})(Submit)
|