mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
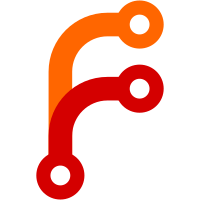
* implemented example for using redux with reselect and recompose * removed unused package * fixed linting issue * fixed linting issue
31 lines
776 B
JavaScript
31 lines
776 B
JavaScript
import { startClock, addCount, serverRenderClock } from 'actions'
|
|
import Page from 'containers/page'
|
|
import withRedux from 'next-redux-wrapper'
|
|
import { compose, setDisplayName, pure, lifecycle, withProps } from 'recompose'
|
|
import initStore from '../store'
|
|
|
|
const Counter = compose(
|
|
setDisplayName('OtherPage'),
|
|
withProps({
|
|
title: 'Other page',
|
|
linkTo: '/'
|
|
}),
|
|
lifecycle({
|
|
componentDidMount () {
|
|
this.timer = this.props.startClock()
|
|
},
|
|
componentWillUnmount () {
|
|
clearInterval(this.timer)
|
|
}
|
|
}),
|
|
pure
|
|
)(Page)
|
|
|
|
Counter.getInitialProps = ({ store, isServer }) => {
|
|
store.dispatch(serverRenderClock(isServer))
|
|
store.dispatch(addCount())
|
|
return { isServer }
|
|
}
|
|
|
|
export default withRedux(initStore, null, { startClock })(Counter)
|