mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
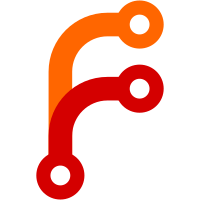
With a hard coded address on the client, the app no longer works when deploying, which is annoying since there's a handy 'deploy now' button on the readme. By removing the hard coded address socket.io will connect to the location host automatically so it works both on development and production.
89 lines
2 KiB
JavaScript
89 lines
2 KiB
JavaScript
import { Component } from 'react'
|
|
import io from 'socket.io-client'
|
|
import fetch from 'isomorphic-fetch'
|
|
|
|
class HomePage extends Component {
|
|
// fetch old messages data from the server
|
|
static async getInitialProps ({ req }) {
|
|
const response = await fetch('http://localhost:3000/messages')
|
|
const messages = await response.json()
|
|
return { messages }
|
|
}
|
|
|
|
static defaultProps = {
|
|
messages: []
|
|
}
|
|
|
|
// init state with the prefetched messages
|
|
state = {
|
|
field: '',
|
|
messages: this.props.messages
|
|
}
|
|
|
|
// connect to WS server and listen event
|
|
componentDidMount () {
|
|
this.socket = io()
|
|
this.socket.on('message', this.handleMessage)
|
|
}
|
|
|
|
// close socket connection
|
|
componentWillUnmount () {
|
|
this.socket.off('message', this.handleMessage)
|
|
this.socket.close()
|
|
}
|
|
|
|
// add messages from server to the state
|
|
handleMessage = (message) => {
|
|
this.setState(state => ({ messages: state.messages.concat(message) }))
|
|
}
|
|
|
|
handleChange = event => {
|
|
this.setState({ field: event.target.value })
|
|
}
|
|
|
|
// send messages to server and add them to the state
|
|
handleSubmit = event => {
|
|
event.preventDefault()
|
|
|
|
// create message object
|
|
const message = {
|
|
id: (new Date()).getTime(),
|
|
value: this.state.field
|
|
}
|
|
|
|
// send object to WS server
|
|
this.socket.emit('message', message)
|
|
|
|
// add it to state and clean current input value
|
|
this.setState(state => ({
|
|
field: '',
|
|
messages: state.messages.concat(message)
|
|
}))
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<main>
|
|
<div>
|
|
<ul>
|
|
{this.state.messages.map(message =>
|
|
<li key={message.id}>{message.value}</li>
|
|
)}
|
|
</ul>
|
|
<form onSubmit={this.handleSubmit}>
|
|
<input
|
|
onChange={this.handleChange}
|
|
type='text'
|
|
placeholder='Hello world!'
|
|
value={this.state.field}
|
|
/>
|
|
<button>Send</button>
|
|
</form>
|
|
</div>
|
|
</main>
|
|
)
|
|
}
|
|
}
|
|
|
|
export default HomePage
|