mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
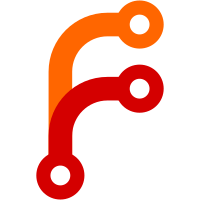
* Simplify route info handling. * Add basic resolve=false support. * Make sure to render getInitialProps always if it's the first render. * Change resolve=false to shallow routing. * Add test cases for shallow routing. * Update README for shallow routing docs. * Update docs. * Update docs. * Update docs.
47 lines
1.2 KiB
JavaScript
47 lines
1.2 KiB
JavaScript
import React from 'react'
|
|
import Link from 'next/link'
|
|
import Router from 'next/router'
|
|
import { format } from 'url'
|
|
|
|
let counter = 1
|
|
|
|
export default class Index extends React.Component {
|
|
static getInitialProps ({ res }) {
|
|
if (res) {
|
|
return { initialPropsCounter: 1 }
|
|
}
|
|
|
|
counter++
|
|
return {
|
|
initialPropsCounter: counter
|
|
}
|
|
}
|
|
|
|
reload () {
|
|
const { pathname, query } = Router
|
|
Router.push(format({ pathname, query }))
|
|
}
|
|
|
|
incrementStateCounter () {
|
|
const { url } = this.props
|
|
const currentCounter = url.query.counter ? parseInt(url.query.counter) : 0
|
|
const href = `/?counter=${currentCounter + 1}`
|
|
Router.push(href, href, { shallow: true })
|
|
}
|
|
|
|
render () {
|
|
const { initialPropsCounter, url } = this.props
|
|
|
|
return (
|
|
<div>
|
|
<h2>This is the Home Page</h2>
|
|
<Link href='/about'><a>About</a></Link>
|
|
<button onClick={() => this.reload()}>Reload</button>
|
|
<button onClick={() => this.incrementStateCounter()}>Change State Counter</button>
|
|
<p>"getInitialProps" ran for "{initialPropsCounter}" times.</p>
|
|
<p>Counter: "{url.query.counter || 0}".</p>
|
|
</div>
|
|
)
|
|
}
|
|
}
|