mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
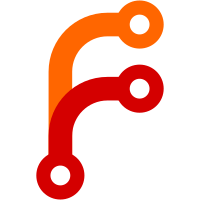
* add cli usage: `next init` * add cli usage: `next --help` * add cli usage: `next build --help` * add cli usage: `next dev --help` * add cli usage: `next init --help` * add cli usage: `next start --help` * use set * build, start and dev all accept a directory * typo and conciseness
51 lines
1 KiB
JavaScript
Executable file
51 lines
1 KiB
JavaScript
Executable file
#!/usr/bin/env node
|
|
|
|
import { resolve } from 'path'
|
|
import parseArgs from 'minimist'
|
|
import Server from '../server'
|
|
|
|
const argv = parseArgs(process.argv.slice(2), {
|
|
alias: {
|
|
h: 'help',
|
|
p: 'port'
|
|
},
|
|
boolean: ['h'],
|
|
default: {
|
|
p: 3000
|
|
}
|
|
})
|
|
|
|
if (argv.help) {
|
|
console.log(`
|
|
Description
|
|
Starts the application in production mode.
|
|
The application should be compiled with \`next build\` first.
|
|
|
|
Usage
|
|
$ next start <dir> -p <port>
|
|
|
|
<dir> is the directory that contains the compiled .next folder
|
|
created by running \`next build\`.
|
|
If no directory is provided, the current directory will be assumed.
|
|
|
|
Options
|
|
--port, -p A port number on which to start the application
|
|
--help, -p Displays this message
|
|
`)
|
|
process.exit(0)
|
|
}
|
|
|
|
const dir = resolve(argv._[0] || '.')
|
|
|
|
const srv = new Server({ dir })
|
|
srv.start(argv.port)
|
|
.then(() => {
|
|
if (!process.env.NOW) {
|
|
console.log(`> Ready on http://localhost:${argv.port}`)
|
|
}
|
|
})
|
|
.catch((err) => {
|
|
console.error(err)
|
|
process.exit(1)
|
|
})
|