mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
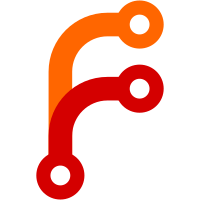
A follow-up to #4604: the dot in the regexp was not escaped as intended. By default `*` matches greedily, so the results are the same, but the new regexp should be more clear. Sorry for the mistake.
63 lines
1.5 KiB
JavaScript
63 lines
1.5 KiB
JavaScript
import { join } from 'path'
|
|
import { readdirSync, existsSync } from 'fs'
|
|
|
|
export function getChunkNameFromFilename (filename, dev) {
|
|
if (dev) {
|
|
return filename.replace(/\.[^.]*$/, '')
|
|
}
|
|
return filename.replace(/-[^-]*$/, '')
|
|
}
|
|
|
|
export function getAvailableChunks (distDir, dev) {
|
|
const chunksDir = join(distDir, 'chunks')
|
|
if (!existsSync(chunksDir)) return {}
|
|
|
|
const chunksMap = {}
|
|
const chunkFiles = readdirSync(chunksDir)
|
|
|
|
chunkFiles.forEach(filename => {
|
|
if (/\.js$/.test(filename)) {
|
|
const chunkName = getChunkNameFromFilename(filename, dev)
|
|
chunksMap[chunkName] = filename
|
|
}
|
|
})
|
|
|
|
return chunksMap
|
|
}
|
|
|
|
const internalPrefixes = [
|
|
/^\/_next\//,
|
|
/^\/static\//
|
|
]
|
|
|
|
export function isInternalUrl (url) {
|
|
for (const prefix of internalPrefixes) {
|
|
if (prefix.test(url)) {
|
|
return true
|
|
}
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
export function addCorsSupport (req, res) {
|
|
if (!req.headers.origin) {
|
|
return { preflight: false }
|
|
}
|
|
|
|
res.setHeader('Access-Control-Allow-Origin', req.headers.origin)
|
|
res.setHeader('Access-Control-Allow-Methods', 'OPTIONS, GET')
|
|
// Based on https://github.com/primus/access-control/blob/4cf1bc0e54b086c91e6aa44fb14966fa5ef7549c/index.js#L158
|
|
if (req.headers['access-control-request-headers']) {
|
|
res.setHeader('Access-Control-Allow-Headers', req.headers['access-control-request-headers'])
|
|
}
|
|
|
|
if (req.method === 'OPTIONS') {
|
|
res.writeHead(200)
|
|
res.end()
|
|
return { preflight: true }
|
|
}
|
|
|
|
return { preflight: false }
|
|
}
|