mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
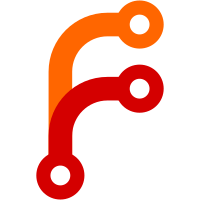
**Changes:** - Fixed "was character fetched on server" message by properly passing `isServer`. - Stop fetching if there was an error (currently it keeps sending requests to the same endpoint every 3 sec) **Related:** - https://github.com/zeit/next.js/pull/4818 - https://github.com/zeit/next.js/issues/4724
52 lines
1.4 KiB
JavaScript
52 lines
1.4 KiB
JavaScript
import { interval, of } from 'rxjs'
|
|
import { takeUntil, mergeMap, catchError, map } from 'rxjs/operators'
|
|
import { combineEpics, ofType } from 'redux-observable'
|
|
import { request } from 'universal-rxjs-ajax' // because standard AjaxObservable only works in browser
|
|
|
|
import * as actions from './actions'
|
|
import * as types from './actionTypes'
|
|
|
|
export const fetchUserEpic = (action$, state$) =>
|
|
action$.pipe(
|
|
ofType(types.START_FETCHING_CHARACTERS),
|
|
mergeMap(action => {
|
|
return interval(3000).pipe(
|
|
map(x => actions.fetchCharacter()),
|
|
takeUntil(action$.ofType(
|
|
types.STOP_FETCHING_CHARACTERS,
|
|
types.FETCH_CHARACTER_FAILURE
|
|
))
|
|
)
|
|
})
|
|
)
|
|
|
|
export const fetchCharacterEpic = (action$, state$) =>
|
|
action$.pipe(
|
|
ofType(types.FETCH_CHARACTER),
|
|
mergeMap(action =>
|
|
request({
|
|
url: `https://swapi.co/api/people/${state$.value.nextCharacterId}`
|
|
}).pipe(
|
|
map(response =>
|
|
actions.fetchCharacterSuccess(
|
|
response.response,
|
|
action.payload.isServer
|
|
)
|
|
),
|
|
catchError(error =>
|
|
of(
|
|
actions.fetchCharacterFailure(
|
|
error.xhr.response,
|
|
action.payload.isServer
|
|
)
|
|
)
|
|
)
|
|
)
|
|
)
|
|
)
|
|
|
|
export const rootEpic = combineEpics(
|
|
fetchUserEpic,
|
|
fetchCharacterEpic
|
|
)
|