mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
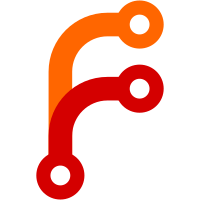
* link: support relative urls * link: support protocol-relative urls * router: fix error for clicking anchor tag
85 lines
2 KiB
JavaScript
85 lines
2 KiB
JavaScript
import { resolve } from 'url'
|
|
import React, { Component, Children, PropTypes } from 'react'
|
|
import Router from './router'
|
|
|
|
export default class Link extends Component {
|
|
constructor (props) {
|
|
super(props)
|
|
this.linkClicked = this.linkClicked.bind(this)
|
|
}
|
|
|
|
static propTypes = {
|
|
children: PropTypes.oneOfType([
|
|
PropTypes.string,
|
|
PropTypes.element
|
|
]).isRequired
|
|
}
|
|
|
|
linkClicked (e) {
|
|
if (e.target.nodeName === 'A' &&
|
|
(e.metaKey || e.ctrlKey || e.shiftKey || (e.nativeEvent && e.nativeEvent.which === 2))) {
|
|
// ignore click for new tab / new window behavior
|
|
return
|
|
}
|
|
|
|
let { href, as } = this.props
|
|
|
|
if (!isLocal(href)) {
|
|
// ignore click if it's outside our scope
|
|
return
|
|
}
|
|
|
|
const { pathname } = window.location
|
|
href = resolve(pathname, href)
|
|
as = as ? resolve(pathname, as) : href
|
|
|
|
e.preventDefault()
|
|
|
|
// avoid scroll for urls with anchor refs
|
|
let { scroll } = this.props
|
|
if (scroll == null) {
|
|
scroll = as.indexOf('#') < 0
|
|
}
|
|
|
|
// straight up redirect
|
|
Router.push(href, as)
|
|
.then((success) => {
|
|
if (!success) return
|
|
if (scroll) window.scrollTo(0, 0)
|
|
})
|
|
.catch((err) => {
|
|
if (this.props.onError) this.props.onError(err)
|
|
})
|
|
}
|
|
|
|
render () {
|
|
const children = Children.map(this.props.children, (child) => {
|
|
const props = {
|
|
onClick: this.linkClicked
|
|
}
|
|
|
|
const isAnchor = child && child.type === 'a'
|
|
|
|
// if child does not specify a href, specify it
|
|
// so that repetition is not needed by the user
|
|
if (!isAnchor || !('href' in child.props)) {
|
|
props.href = this.props.as || this.props.href
|
|
}
|
|
|
|
if (isAnchor) {
|
|
return React.cloneElement(child, props)
|
|
} else {
|
|
return <a {...props}>{child}</a>
|
|
}
|
|
})
|
|
|
|
return children[0]
|
|
}
|
|
}
|
|
|
|
export function isLocal (href) {
|
|
const origin = window.location.origin
|
|
return !/^(https?:)?\/\//.test(href) ||
|
|
origin === href.substr(0, origin.length)
|
|
}
|