mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
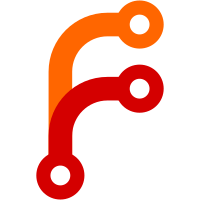
* Implement a very simple prefetching solution. * Remove next-prefetcher. * Require 'whatwg-fetch' only in the client. * Use xhr in the code. * Use a simple fetching solution. * Fix 404 and xhr status issue. * Move the prefetching implementation to next/router. * Add deprecated warnning for next/prefetch * Run only 2 parellel prefetching request at a time. * Change xhr to jsonPageRes. * Improve the prefetching logic. * Add unit tests covering the Router.prefetch() * Update examples to use the new syntax. * Update docs. * Use execOnce() to manage warn printing. * Remove prefetcher building from the flyfile.js Because, we no longer use it.
89 lines
1.9 KiB
JavaScript
89 lines
1.9 KiB
JavaScript
export function warn (message) {
|
|
if (process.env.NODE_ENV !== 'production') {
|
|
console.error(message)
|
|
}
|
|
}
|
|
|
|
export function execOnce (fn) {
|
|
let used = false
|
|
return (...args) => {
|
|
if (!used) {
|
|
used = true
|
|
fn.apply(this, args)
|
|
}
|
|
}
|
|
}
|
|
|
|
export function deprecated (fn, message) {
|
|
if (process.env.NODE_ENV === 'production') return fn
|
|
|
|
let warned = false
|
|
const newFn = function (...args) {
|
|
if (!warned) {
|
|
warned = true
|
|
console.error(message)
|
|
}
|
|
return fn.apply(this, args)
|
|
}
|
|
|
|
// copy all properties
|
|
Object.assign(newFn, fn)
|
|
|
|
return newFn
|
|
}
|
|
|
|
export function printAndExit (message, code = 1) {
|
|
if (code === 0) {
|
|
console.log(message)
|
|
} else {
|
|
console.error(message)
|
|
}
|
|
|
|
process.exit(code)
|
|
}
|
|
|
|
export async function loadGetInitialProps (Component, ctx) {
|
|
if (!Component.getInitialProps) return {}
|
|
|
|
const props = await Component.getInitialProps(ctx)
|
|
if (!props && (!ctx.res || !ctx.res.finished)) {
|
|
const compName = Component.displayName || Component.name
|
|
const message = `"${compName}.getInitialProps()" should resolve to an object. But found "${props}" instead.`
|
|
throw new Error(message)
|
|
}
|
|
return props
|
|
}
|
|
|
|
export class LockManager {
|
|
constructor (maxLocks) {
|
|
this.maxLocks = maxLocks
|
|
this.clients = []
|
|
this.runningLocks = 0
|
|
}
|
|
|
|
get () {
|
|
return new Promise((resolve) => {
|
|
this.clients.push(resolve)
|
|
this._giveLocksIfPossible()
|
|
})
|
|
}
|
|
|
|
_giveLocksIfPossible () {
|
|
if (this.runningLocks < this.maxLocks) {
|
|
const client = this.clients.shift()
|
|
if (!client) return
|
|
|
|
this.runningLocks ++
|
|
client(() => {
|
|
this.runningLocks --
|
|
this._giveLocksIfPossible()
|
|
})
|
|
}
|
|
}
|
|
}
|
|
|
|
export function getLocationOrigin () {
|
|
const { protocol, hostname, port } = window.location
|
|
return `${protocol}//${hostname}${port ? ':' + port : ''}`
|
|
}
|