mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
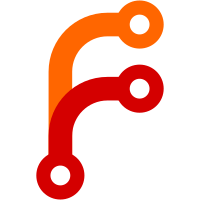
* Add prettier for examples directory * Fix files * Fix linting * Add prettier script in case it has to be ran again
54 lines
1.2 KiB
JavaScript
54 lines
1.2 KiB
JavaScript
import React from 'react'
|
|
import { bindActionCreators } from 'redux'
|
|
import {
|
|
initStore,
|
|
startClock,
|
|
addCount,
|
|
serverRenderClock
|
|
} from '../lib/store'
|
|
import withRedux from 'next-redux-wrapper'
|
|
|
|
import App from '../components/App'
|
|
import Header from '../components/Header'
|
|
import Page from '../components/Page'
|
|
import Submit from '../components/Submit'
|
|
import PostList from '../components/PostList'
|
|
import withApollo from '../lib/withApollo'
|
|
|
|
class Index extends React.Component {
|
|
static getInitialProps ({ store, isServer }) {
|
|
store.dispatch(serverRenderClock(isServer))
|
|
store.dispatch(addCount())
|
|
|
|
return { isServer }
|
|
}
|
|
|
|
componentDidMount () {
|
|
this.timer = this.props.startClock()
|
|
}
|
|
|
|
componentWillUnmount () {
|
|
clearInterval(this.timer)
|
|
}
|
|
|
|
render () {
|
|
return (
|
|
<App>
|
|
<Header />
|
|
<Page title='Index' />
|
|
<Submit />
|
|
<PostList />
|
|
</App>
|
|
)
|
|
}
|
|
}
|
|
|
|
const mapDispatchToProps = dispatch => {
|
|
return {
|
|
addCount: bindActionCreators(addCount, dispatch),
|
|
startClock: bindActionCreators(startClock, dispatch)
|
|
}
|
|
}
|
|
|
|
export default withApollo(withRedux(initStore, null, mapDispatchToProps)(Index))
|