mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
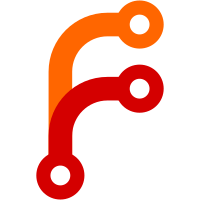
glide has its own requirements. My previous workaround caused me some code checkin errors. Need to fix this.
66 lines
1.2 KiB
Go
66 lines
1.2 KiB
Go
package topology
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
type VolumeLocationList struct {
|
|
list []*DataNode
|
|
}
|
|
|
|
func NewVolumeLocationList() *VolumeLocationList {
|
|
return &VolumeLocationList{}
|
|
}
|
|
|
|
func (dnll *VolumeLocationList) String() string {
|
|
return fmt.Sprintf("%v", dnll.list)
|
|
}
|
|
|
|
func (dnll *VolumeLocationList) Head() *DataNode {
|
|
//mark first node as master volume
|
|
return dnll.list[0]
|
|
}
|
|
|
|
func (dnll *VolumeLocationList) Length() int {
|
|
return len(dnll.list)
|
|
}
|
|
|
|
func (dnll *VolumeLocationList) Set(loc *DataNode) {
|
|
for i := 0; i < len(dnll.list); i++ {
|
|
if loc.Ip == dnll.list[i].Ip && loc.Port == dnll.list[i].Port {
|
|
dnll.list[i] = loc
|
|
return
|
|
}
|
|
}
|
|
dnll.list = append(dnll.list, loc)
|
|
}
|
|
|
|
func (dnll *VolumeLocationList) Remove(loc *DataNode) bool {
|
|
for i, dnl := range dnll.list {
|
|
if loc.Ip == dnl.Ip && loc.Port == dnl.Port {
|
|
dnll.list = append(dnll.list[:i], dnll.list[i+1:]...)
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (dnll *VolumeLocationList) Refresh(freshThreshHold int64) {
|
|
var changed bool
|
|
for _, dnl := range dnll.list {
|
|
if dnl.LastSeen < freshThreshHold {
|
|
changed = true
|
|
break
|
|
}
|
|
}
|
|
if changed {
|
|
var l []*DataNode
|
|
for _, dnl := range dnll.list {
|
|
if dnl.LastSeen >= freshThreshHold {
|
|
l = append(l, dnl)
|
|
}
|
|
}
|
|
dnll.list = l
|
|
}
|
|
}
|