mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
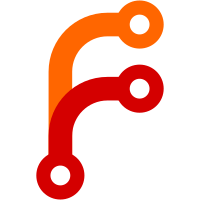
glide has its own requirements. My previous workaround caused me some code checkin errors. Need to fix this.
65 lines
2.1 KiB
Go
65 lines
2.1 KiB
Go
package weed_server
|
|
|
|
import (
|
|
"encoding/json"
|
|
"io/ioutil"
|
|
"net/http"
|
|
"strings"
|
|
|
|
"github.com/chrislusf/raft"
|
|
"github.com/chrislusf/seaweedfs/weed/glog"
|
|
"github.com/chrislusf/seaweedfs/weed/operation"
|
|
)
|
|
|
|
// Handles incoming RAFT joins.
|
|
func (s *RaftServer) joinHandler(w http.ResponseWriter, req *http.Request) {
|
|
glog.V(0).Infoln("Processing incoming join. Current Leader", s.raftServer.Leader(), "Self", s.raftServer.Name(), "Peers", s.raftServer.Peers())
|
|
command := &raft.DefaultJoinCommand{}
|
|
|
|
commandText, _ := ioutil.ReadAll(req.Body)
|
|
glog.V(0).Info("Command:", string(commandText))
|
|
if err := json.NewDecoder(strings.NewReader(string(commandText))).Decode(&command); err != nil {
|
|
glog.V(0).Infoln("Error decoding json message:", err, string(commandText))
|
|
http.Error(w, err.Error(), http.StatusInternalServerError)
|
|
return
|
|
}
|
|
|
|
glog.V(0).Infoln("join command from Name", command.Name, "Connection", command.ConnectionString)
|
|
|
|
if _, err := s.raftServer.Do(command); err != nil {
|
|
switch err {
|
|
case raft.NotLeaderError:
|
|
s.redirectToLeader(w, req)
|
|
default:
|
|
glog.V(0).Infoln("Error processing join:", err)
|
|
http.Error(w, err.Error(), http.StatusInternalServerError)
|
|
}
|
|
}
|
|
}
|
|
|
|
func (s *RaftServer) HandleFunc(pattern string, handler func(http.ResponseWriter, *http.Request)) {
|
|
s.router.HandleFunc(pattern, handler)
|
|
}
|
|
|
|
func (s *RaftServer) redirectToLeader(w http.ResponseWriter, req *http.Request) {
|
|
if leader, e := s.topo.Leader(); e == nil {
|
|
//http.StatusMovedPermanently does not cause http POST following redirection
|
|
glog.V(0).Infoln("Redirecting to", http.StatusMovedPermanently, "http://"+leader+req.URL.Path)
|
|
http.Redirect(w, req, "http://"+leader+req.URL.Path, http.StatusMovedPermanently)
|
|
} else {
|
|
glog.V(0).Infoln("Error: Leader Unknown")
|
|
http.Error(w, "Leader unknown", http.StatusInternalServerError)
|
|
}
|
|
}
|
|
|
|
func (s *RaftServer) statusHandler(w http.ResponseWriter, r *http.Request) {
|
|
ret := operation.ClusterStatusResult{
|
|
IsLeader: s.topo.IsLeader(),
|
|
Peers: s.Peers(),
|
|
}
|
|
if leader, e := s.topo.Leader(); e == nil {
|
|
ret.Leader = leader
|
|
}
|
|
writeJsonQuiet(w, r, http.StatusOK, ret)
|
|
}
|