mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
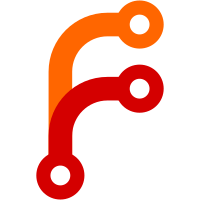
glide has its own requirements. My previous workaround caused me some code checkin errors. Need to fix this.
97 lines
2.6 KiB
Go
97 lines
2.6 KiB
Go
package operation
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/json"
|
|
"errors"
|
|
"fmt"
|
|
"io"
|
|
"io/ioutil"
|
|
"mime"
|
|
"mime/multipart"
|
|
"net/http"
|
|
"net/textproto"
|
|
"path/filepath"
|
|
"strings"
|
|
|
|
"github.com/chrislusf/seaweedfs/weed/glog"
|
|
"github.com/chrislusf/seaweedfs/weed/security"
|
|
)
|
|
|
|
type UploadResult struct {
|
|
Name string `json:"name,omitempty"`
|
|
Size uint32 `json:"size,omitempty"`
|
|
Error string `json:"error,omitempty"`
|
|
}
|
|
|
|
var (
|
|
client *http.Client
|
|
)
|
|
|
|
func init() {
|
|
client = &http.Client{Transport: &http.Transport{
|
|
MaxIdleConnsPerHost: 1024,
|
|
}}
|
|
}
|
|
|
|
var fileNameEscaper = strings.NewReplacer("\\", "\\\\", "\"", "\\\"")
|
|
|
|
func Upload(uploadUrl string, filename string, reader io.Reader, isGzipped bool, mtype string, jwt security.EncodedJwt) (*UploadResult, error) {
|
|
return upload_content(uploadUrl, func(w io.Writer) (err error) {
|
|
_, err = io.Copy(w, reader)
|
|
return
|
|
}, filename, isGzipped, mtype, jwt)
|
|
}
|
|
func upload_content(uploadUrl string, fillBufferFunction func(w io.Writer) error, filename string, isGzipped bool, mtype string, jwt security.EncodedJwt) (*UploadResult, error) {
|
|
body_buf := bytes.NewBufferString("")
|
|
body_writer := multipart.NewWriter(body_buf)
|
|
h := make(textproto.MIMEHeader)
|
|
h.Set("Content-Disposition", fmt.Sprintf(`form-data; name="file"; filename="%s"`, fileNameEscaper.Replace(filename)))
|
|
if mtype == "" {
|
|
mtype = mime.TypeByExtension(strings.ToLower(filepath.Ext(filename)))
|
|
}
|
|
if mtype != "" {
|
|
h.Set("Content-Type", mtype)
|
|
}
|
|
if isGzipped {
|
|
h.Set("Content-Encoding", "gzip")
|
|
}
|
|
if jwt != "" {
|
|
h.Set("Authorization", "BEARER "+string(jwt))
|
|
}
|
|
file_writer, cp_err := body_writer.CreatePart(h)
|
|
if cp_err != nil {
|
|
glog.V(0).Infoln("error creating form file", cp_err.Error())
|
|
return nil, cp_err
|
|
}
|
|
if err := fillBufferFunction(file_writer); err != nil {
|
|
glog.V(0).Infoln("error copying data", err)
|
|
return nil, err
|
|
}
|
|
content_type := body_writer.FormDataContentType()
|
|
if err := body_writer.Close(); err != nil {
|
|
glog.V(0).Infoln("error closing body", err)
|
|
return nil, err
|
|
}
|
|
resp, post_err := client.Post(uploadUrl, content_type, body_buf)
|
|
if post_err != nil {
|
|
glog.V(0).Infoln("failing to upload to", uploadUrl, post_err.Error())
|
|
return nil, post_err
|
|
}
|
|
defer resp.Body.Close()
|
|
resp_body, ra_err := ioutil.ReadAll(resp.Body)
|
|
if ra_err != nil {
|
|
return nil, ra_err
|
|
}
|
|
var ret UploadResult
|
|
unmarshal_err := json.Unmarshal(resp_body, &ret)
|
|
if unmarshal_err != nil {
|
|
glog.V(0).Infoln("failing to read upload resonse", uploadUrl, string(resp_body))
|
|
return nil, unmarshal_err
|
|
}
|
|
if ret.Error != "" {
|
|
return nil, errors.New(ret.Error)
|
|
}
|
|
return &ret, nil
|
|
}
|