mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
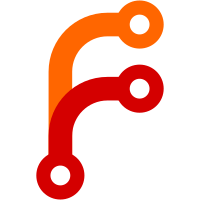
glide has its own requirements. My previous workaround caused me some code checkin errors. Need to fix this.
33 lines
749 B
Go
33 lines
749 B
Go
package compress
|
|
|
|
import (
|
|
"github.com/reducedb/encoding/cursor"
|
|
"github.com/reducedb/encoding/delta/bp32"
|
|
)
|
|
|
|
// Compress compresses in[]int32 to out[]int32
|
|
func Compress32(in []int32) (out []int32, err error) {
|
|
out = make([]int32, len(in)*2)
|
|
inpos := cursor.New()
|
|
outpos := cursor.New()
|
|
|
|
if err = bp32.New().Compress(in, inpos, len(in), out, outpos); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return out[:outpos.Get()], nil
|
|
}
|
|
|
|
// Uncompress uncompresses in[]int32 to out[]int32
|
|
func Uncompress32(in []int32, buffer []int32) (out []int32, err error) {
|
|
out = buffer
|
|
inpos := cursor.New()
|
|
outpos := cursor.New()
|
|
|
|
if err = bp32.New().Uncompress(in, inpos, len(in), out, outpos); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return out[:outpos.Get()], nil
|
|
}
|