mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
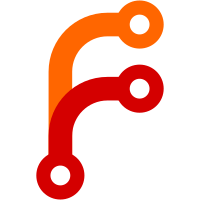
streaming mode would create separate grpc connections for each call. this is to ensure the long poll connections are properly closed.
58 lines
1.1 KiB
Go
58 lines
1.1 KiB
Go
package shell
|
|
|
|
import (
|
|
"context"
|
|
"github.com/chrislusf/seaweedfs/weed/pb/filer_pb"
|
|
"github.com/chrislusf/seaweedfs/weed/util"
|
|
"io"
|
|
"os"
|
|
"time"
|
|
)
|
|
|
|
func init() {
|
|
Commands = append(Commands, &commandFsMkdir{})
|
|
}
|
|
|
|
type commandFsMkdir struct {
|
|
}
|
|
|
|
func (c *commandFsMkdir) Name() string {
|
|
return "fs.mkdir"
|
|
}
|
|
|
|
func (c *commandFsMkdir) Help() string {
|
|
return `create a directory
|
|
|
|
fs.mkdir path/to/dir
|
|
`
|
|
}
|
|
|
|
func (c *commandFsMkdir) Do(args []string, commandEnv *CommandEnv, writer io.Writer) (err error) {
|
|
|
|
path, err := commandEnv.parseUrl(findInputDirectory(args))
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
dir, name := util.FullPath(path).DirAndName()
|
|
|
|
err = commandEnv.WithFilerClient(false, func(client filer_pb.SeaweedFilerClient) error {
|
|
|
|
_, createErr := client.CreateEntry(context.Background(), &filer_pb.CreateEntryRequest{
|
|
Directory: dir,
|
|
Entry: &filer_pb.Entry{
|
|
Name: name,
|
|
IsDirectory: true,
|
|
Attributes: &filer_pb.FuseAttributes{
|
|
Mtime: time.Now().Unix(),
|
|
Crtime: time.Now().Unix(),
|
|
FileMode: uint32(0777 | os.ModeDir),
|
|
},
|
|
},
|
|
})
|
|
return createErr
|
|
})
|
|
|
|
return
|
|
}
|