mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
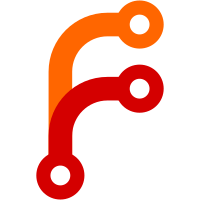
glide has its own requirements. My previous workaround caused me some code checkin errors. Need to fix this.
171 lines
3.2 KiB
Go
171 lines
3.2 KiB
Go
package main
|
|
|
|
import (
|
|
"flag"
|
|
"fmt"
|
|
"io"
|
|
"math/rand"
|
|
"os"
|
|
"strings"
|
|
"sync"
|
|
"text/template"
|
|
"time"
|
|
"unicode"
|
|
"unicode/utf8"
|
|
|
|
"github.com/chrislusf/seaweedfs/weed/command"
|
|
"github.com/chrislusf/seaweedfs/weed/glog"
|
|
)
|
|
|
|
var IsDebug *bool
|
|
var server *string
|
|
|
|
var commands = command.Commands
|
|
|
|
var exitStatus = 0
|
|
var exitMu sync.Mutex
|
|
|
|
func setExitStatus(n int) {
|
|
exitMu.Lock()
|
|
if exitStatus < n {
|
|
exitStatus = n
|
|
}
|
|
exitMu.Unlock()
|
|
}
|
|
|
|
func main() {
|
|
glog.MaxSize = 1024 * 1024 * 32
|
|
rand.Seed(time.Now().UnixNano())
|
|
flag.Usage = usage
|
|
flag.Parse()
|
|
|
|
args := flag.Args()
|
|
if len(args) < 1 {
|
|
usage()
|
|
}
|
|
|
|
if args[0] == "help" {
|
|
help(args[1:])
|
|
for _, cmd := range commands {
|
|
if len(args) >= 2 && cmd.Name() == args[1] && cmd.Run != nil {
|
|
fmt.Fprintf(os.Stderr, "Default Parameters:\n")
|
|
cmd.Flag.PrintDefaults()
|
|
}
|
|
}
|
|
return
|
|
}
|
|
|
|
for _, cmd := range commands {
|
|
if cmd.Name() == args[0] && cmd.Run != nil {
|
|
cmd.Flag.Usage = func() { cmd.Usage() }
|
|
cmd.Flag.Parse(args[1:])
|
|
args = cmd.Flag.Args()
|
|
IsDebug = cmd.IsDebug
|
|
if !cmd.Run(cmd, args) {
|
|
fmt.Fprintf(os.Stderr, "\n")
|
|
cmd.Flag.Usage()
|
|
fmt.Fprintf(os.Stderr, "Default Parameters:\n")
|
|
cmd.Flag.PrintDefaults()
|
|
}
|
|
exit()
|
|
return
|
|
}
|
|
}
|
|
|
|
fmt.Fprintf(os.Stderr, "weed: unknown subcommand %q\nRun 'weed help' for usage.\n", args[0])
|
|
setExitStatus(2)
|
|
exit()
|
|
}
|
|
|
|
var usageTemplate = `
|
|
SeaweedFS: store billions of files and serve them fast!
|
|
|
|
Usage:
|
|
|
|
weed command [arguments]
|
|
|
|
The commands are:
|
|
{{range .}}{{if .Runnable}}
|
|
{{.Name | printf "%-11s"}} {{.Short}}{{end}}{{end}}
|
|
|
|
Use "weed help [command]" for more information about a command.
|
|
|
|
`
|
|
|
|
var helpTemplate = `{{if .Runnable}}Usage: weed {{.UsageLine}}
|
|
{{end}}
|
|
{{.Long}}
|
|
`
|
|
|
|
// tmpl executes the given template text on data, writing the result to w.
|
|
func tmpl(w io.Writer, text string, data interface{}) {
|
|
t := template.New("top")
|
|
t.Funcs(template.FuncMap{"trim": strings.TrimSpace, "capitalize": capitalize})
|
|
template.Must(t.Parse(text))
|
|
if err := t.Execute(w, data); err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
func capitalize(s string) string {
|
|
if s == "" {
|
|
return s
|
|
}
|
|
r, n := utf8.DecodeRuneInString(s)
|
|
return string(unicode.ToTitle(r)) + s[n:]
|
|
}
|
|
|
|
func printUsage(w io.Writer) {
|
|
tmpl(w, usageTemplate, commands)
|
|
}
|
|
|
|
func usage() {
|
|
printUsage(os.Stderr)
|
|
fmt.Fprintf(os.Stderr, "For Logging, use \"weed [logging_options] [command]\". The logging options are:\n")
|
|
flag.PrintDefaults()
|
|
os.Exit(2)
|
|
}
|
|
|
|
// help implements the 'help' command.
|
|
func help(args []string) {
|
|
if len(args) == 0 {
|
|
printUsage(os.Stdout)
|
|
// not exit 2: succeeded at 'weed help'.
|
|
return
|
|
}
|
|
if len(args) != 1 {
|
|
fmt.Fprintf(os.Stderr, "usage: weed help command\n\nToo many arguments given.\n")
|
|
os.Exit(2) // failed at 'weed help'
|
|
}
|
|
|
|
arg := args[0]
|
|
|
|
for _, cmd := range commands {
|
|
if cmd.Name() == arg {
|
|
tmpl(os.Stdout, helpTemplate, cmd)
|
|
// not exit 2: succeeded at 'weed help cmd'.
|
|
return
|
|
}
|
|
}
|
|
|
|
fmt.Fprintf(os.Stderr, "Unknown help topic %#q. Run 'weed help'.\n", arg)
|
|
os.Exit(2) // failed at 'weed help cmd'
|
|
}
|
|
|
|
var atexitFuncs []func()
|
|
|
|
func atexit(f func()) {
|
|
atexitFuncs = append(atexitFuncs, f)
|
|
}
|
|
|
|
func exit() {
|
|
for _, f := range atexitFuncs {
|
|
f()
|
|
}
|
|
os.Exit(exitStatus)
|
|
}
|
|
|
|
func debug(params ...interface{}) {
|
|
glog.V(4).Infoln(params)
|
|
}
|