mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
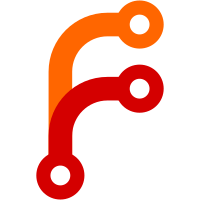
glide has its own requirements. My previous workaround caused me some code checkin errors. Need to fix this.
54 lines
1.3 KiB
Go
54 lines
1.3 KiB
Go
package topology
|
|
|
|
func (t *Topology) ToMap() interface{} {
|
|
m := make(map[string]interface{})
|
|
m["Max"] = t.GetMaxVolumeCount()
|
|
m["Free"] = t.FreeSpace()
|
|
var dcs []interface{}
|
|
for _, c := range t.Children() {
|
|
dc := c.(*DataCenter)
|
|
dcs = append(dcs, dc.ToMap())
|
|
}
|
|
m["DataCenters"] = dcs
|
|
var layouts []interface{}
|
|
for _, col := range t.collectionMap.Items() {
|
|
c := col.(*Collection)
|
|
for _, layout := range c.storageType2VolumeLayout.Items() {
|
|
if layout != nil {
|
|
tmp := layout.(*VolumeLayout).ToMap()
|
|
tmp["collection"] = c.Name
|
|
layouts = append(layouts, tmp)
|
|
}
|
|
}
|
|
}
|
|
m["layouts"] = layouts
|
|
return m
|
|
}
|
|
|
|
func (t *Topology) ToVolumeMap() interface{} {
|
|
m := make(map[string]interface{})
|
|
m["Max"] = t.GetMaxVolumeCount()
|
|
m["Free"] = t.FreeSpace()
|
|
dcs := make(map[NodeId]interface{})
|
|
for _, c := range t.Children() {
|
|
dc := c.(*DataCenter)
|
|
racks := make(map[NodeId]interface{})
|
|
for _, r := range dc.Children() {
|
|
rack := r.(*Rack)
|
|
dataNodes := make(map[NodeId]interface{})
|
|
for _, d := range rack.Children() {
|
|
dn := d.(*DataNode)
|
|
var volumes []interface{}
|
|
for _, v := range dn.GetVolumes() {
|
|
volumes = append(volumes, v)
|
|
}
|
|
dataNodes[d.Id()] = volumes
|
|
}
|
|
racks[r.Id()] = dataNodes
|
|
}
|
|
dcs[dc.Id()] = racks
|
|
}
|
|
m["DataCenters"] = dcs
|
|
return m
|
|
}
|