mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
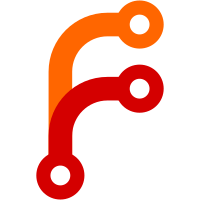
1. switch to viper for filer store configuration 2. simplify FindEntry() return values, removing “found” 3. add leveldb store
62 lines
1.3 KiB
Go
62 lines
1.3 KiB
Go
package leveldb
|
|
|
|
import (
|
|
"testing"
|
|
"github.com/chrislusf/seaweedfs/weed/filer2"
|
|
"io/ioutil"
|
|
"os"
|
|
)
|
|
|
|
func TestCreateAndFind(t *testing.T) {
|
|
filer := filer2.NewFiler("")
|
|
dir, _ := ioutil.TempDir("", "seaweedfs_filer_test")
|
|
defer os.RemoveAll(dir)
|
|
store := &LevelDBStore{}
|
|
store.initialize(dir)
|
|
filer.SetStore(store)
|
|
filer.DisableDirectoryCache()
|
|
|
|
fullpath := filer2.FullPath("/home/chris/this/is/one/file1.jpg")
|
|
|
|
entry1 := &filer2.Entry{
|
|
FullPath: fullpath,
|
|
Attr: filer2.Attr{
|
|
Mode: 0440,
|
|
Uid: 1234,
|
|
Gid: 5678,
|
|
},
|
|
}
|
|
|
|
if err := filer.CreateEntry(entry1); err != nil {
|
|
t.Errorf("create entry %v: %v", entry1.FullPath, err)
|
|
return
|
|
}
|
|
|
|
entry, err := filer.FindEntry(fullpath)
|
|
|
|
if err != nil {
|
|
t.Errorf("find entry: %v", err)
|
|
return
|
|
}
|
|
|
|
if entry.FullPath != entry1.FullPath {
|
|
t.Errorf("find wrong entry: %v", entry.FullPath)
|
|
return
|
|
}
|
|
|
|
// checking one upper directory
|
|
entries, _ := filer.ListDirectoryEntries(filer2.FullPath("/home/chris/this/is/one"), "", false, 100)
|
|
if len(entries) != 1 {
|
|
t.Errorf("list entries count: %v", len(entries))
|
|
return
|
|
}
|
|
|
|
// checking one upper directory
|
|
entries, _ = filer.ListDirectoryEntries(filer2.FullPath("/"), "", false, 100)
|
|
if len(entries) != 1 {
|
|
t.Errorf("list entries count: %v", len(entries))
|
|
return
|
|
}
|
|
|
|
}
|