mirror of
https://github.com/seaweedfs/seaweedfs.git
synced 2024-01-19 02:48:24 +00:00
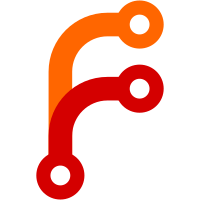
change replication_type to ReplicaPlacement, hopefully cleaner code works for 9 possible ReplicaPlacement xyz x : number of copies on other data centers y : number of copies on other racks z : number of copies on current rack x y z each can be 0,1,2 Minor: weed server "-mdir" default to "-dir" if empty
39 lines
1.1 KiB
Go
39 lines
1.1 KiB
Go
package topology
|
|
|
|
import (
|
|
"code.google.com/p/weed-fs/go/glog"
|
|
"code.google.com/p/weed-fs/go/storage"
|
|
)
|
|
|
|
type Collection struct {
|
|
Name string
|
|
volumeSizeLimit uint64
|
|
replicaType2VolumeLayout []*VolumeLayout
|
|
}
|
|
|
|
func NewCollection(name string, volumeSizeLimit uint64) *Collection {
|
|
c := &Collection{Name: name, volumeSizeLimit: volumeSizeLimit}
|
|
c.replicaType2VolumeLayout = make([]*VolumeLayout, storage.ReplicaPlacementCount)
|
|
return c
|
|
}
|
|
|
|
func (c *Collection) GetOrCreateVolumeLayout(rp *storage.ReplicaPlacement) *VolumeLayout {
|
|
replicaPlacementIndex := rp.GetReplicationLevelIndex()
|
|
if c.replicaType2VolumeLayout[replicaPlacementIndex] == nil {
|
|
glog.V(0).Infoln("collection", c.Name, "adding replication type", rp)
|
|
c.replicaType2VolumeLayout[replicaPlacementIndex] = NewVolumeLayout(rp, c.volumeSizeLimit)
|
|
}
|
|
return c.replicaType2VolumeLayout[replicaPlacementIndex]
|
|
}
|
|
|
|
func (c *Collection) Lookup(vid storage.VolumeId) []*DataNode {
|
|
for _, vl := range c.replicaType2VolumeLayout {
|
|
if vl != nil {
|
|
if list := vl.Lookup(vid); list != nil {
|
|
return list
|
|
}
|
|
}
|
|
}
|
|
return nil
|
|
}
|