mirror of
https://github.com/ytdl-org/youtube-dl.git
synced 2024-01-07 17:16:08 +00:00
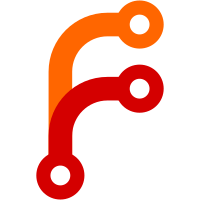
We could only know if we had to delete the original file, but this system allows to specify us more files (like subtitles).
48 lines
1.5 KiB
Python
48 lines
1.5 KiB
Python
from __future__ import unicode_literals
|
|
|
|
import re
|
|
|
|
from .common import PostProcessor
|
|
from ..utils import PostProcessingError
|
|
|
|
|
|
class MetadataFromTitlePPError(PostProcessingError):
|
|
pass
|
|
|
|
|
|
class MetadataFromTitlePP(PostProcessor):
|
|
def __init__(self, downloader, titleformat):
|
|
super(MetadataFromTitlePP, self).__init__(downloader)
|
|
self._titleformat = titleformat
|
|
self._titleregex = self.format_to_regex(titleformat)
|
|
|
|
def format_to_regex(self, fmt):
|
|
"""
|
|
Converts a string like
|
|
'%(title)s - %(artist)s'
|
|
to a regex like
|
|
'(?P<title>.+)\ \-\ (?P<artist>.+)'
|
|
"""
|
|
lastpos = 0
|
|
regex = ""
|
|
# replace %(..)s with regex group and escape other string parts
|
|
for match in re.finditer(r'%\((\w+)\)s', fmt):
|
|
regex += re.escape(fmt[lastpos:match.start()])
|
|
regex += r'(?P<' + match.group(1) + '>.+)'
|
|
lastpos = match.end()
|
|
if lastpos < len(fmt):
|
|
regex += re.escape(fmt[lastpos:len(fmt)])
|
|
return regex
|
|
|
|
def run(self, info):
|
|
title = info['title']
|
|
match = re.match(self._titleregex, title)
|
|
if match is None:
|
|
raise MetadataFromTitlePPError('Could not interpret title of video as "%s"' % self._titleformat)
|
|
for attribute, value in match.groupdict().items():
|
|
value = match.group(attribute)
|
|
info[attribute] = value
|
|
self._downloader.to_screen('[fromtitle] parsed ' + attribute + ': ' + value)
|
|
|
|
return [], info
|